Security Tip: Validating Array Inputs
[Tip#42] Validating single values is easy, but what about arrays?
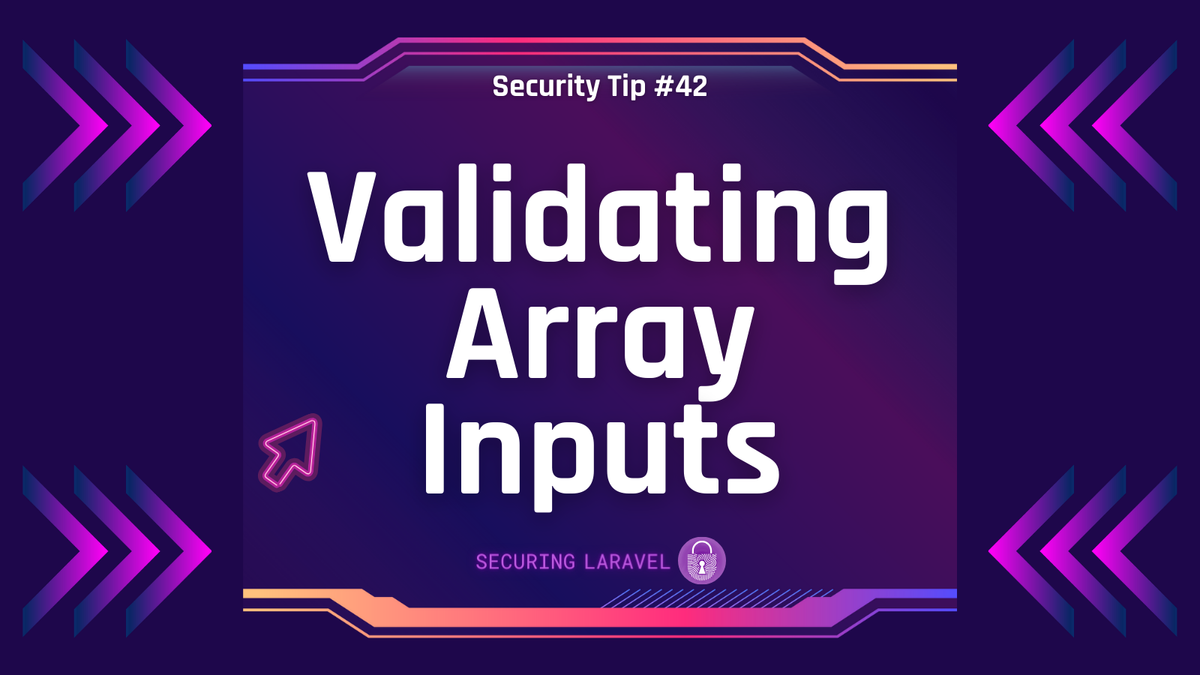
#1 → Exposed API Keys & Passwords
#2 → Missing Authorisation
#3 → Missing Content Security Policy (CSP)
#4 → Missing Security Headers
#5 → Insecure Function Use
#6 → Outdated & Vulnerable Dependencies
#7 → Cross-Site Scripting (XSS)
#8 → Insufficient Rate Limiting
#9 → Missing Subresource Integrity (SRI)
#10 → Insufficient Input Validation & Mass-Assignment Vulnerabilities
A common excuse for not validating inputs is complexity.
When building a complicated interface, it’s easy to get overwhelmed by the number of inputs your passing around, and then adding in arrays - and nested arrays - just bumps up the complexity significantly.
As a developer, I totally get it. I’ve done the same! But as a security person, and a hacker, I see opportunities to inject stuff! 😈
When attacking a form (during an audit), I specifically go looking for nested array inputs, and then see what I can inject into them. I look for sensitive columns, such as users.admin
, and see what it’ll accept. Nested and related models are gold for this, as they are often blindly synced in batch, rather than carefully checked individually.
So how should you validate array inputs?
There are a few options, depending on what you need to do. Let’s take a look at each in turn.
array
validation rule.
It allows you to specify which fields must be present in the input array.$input = [ 'user' => [ 'name' => 'Frodo Baggins', 'sword' => 'Sting', 'ring' => true, ], ]; $request->validate([ 'user' => 'array:name,sword,ring', ]);.
Simple dot-notation for nested values.
Like elsewhere in Laravel, you can use dot-notation to traverse arrays, and assign unique rules to each array item. This is a great approach for ensuring each value is properly validated.$input = [ 'user' => [ 'name' => 'Frodo Baggins', 'sword' => 'Sting', 'ring' => true, ], ]; $request->validate([ 'user.name' => 'required|string', 'user.sword' => 'nullable|string', 'user.ring' => 'required|boolean', ]);.
Wildcard dot-notation for nested arrays.
When dealing with arrays of items nested under a key, you can use the`*`
wildcard to easily validate the keys within the array items.$input = [ 'users' => [ ['name' => 'Frodo Baggins', 'sword' => 'Sting', 'ring' => true], ['name' => 'Gollum', 'sword' => null, 'ring' => false], ], ]; $request->validate([ 'users.*.name' => 'required|string', 'users.*.sword' => 'nullable|string', 'users.*.ring' => 'required|boolean', ]);.