Security Tip: Laravel's Password Generator
[Tip#37] If you need to generate passwords in your app, it's important to use a cryptographically secure algorithm. Laravel makes this easy by giving us the Str::password() helper!
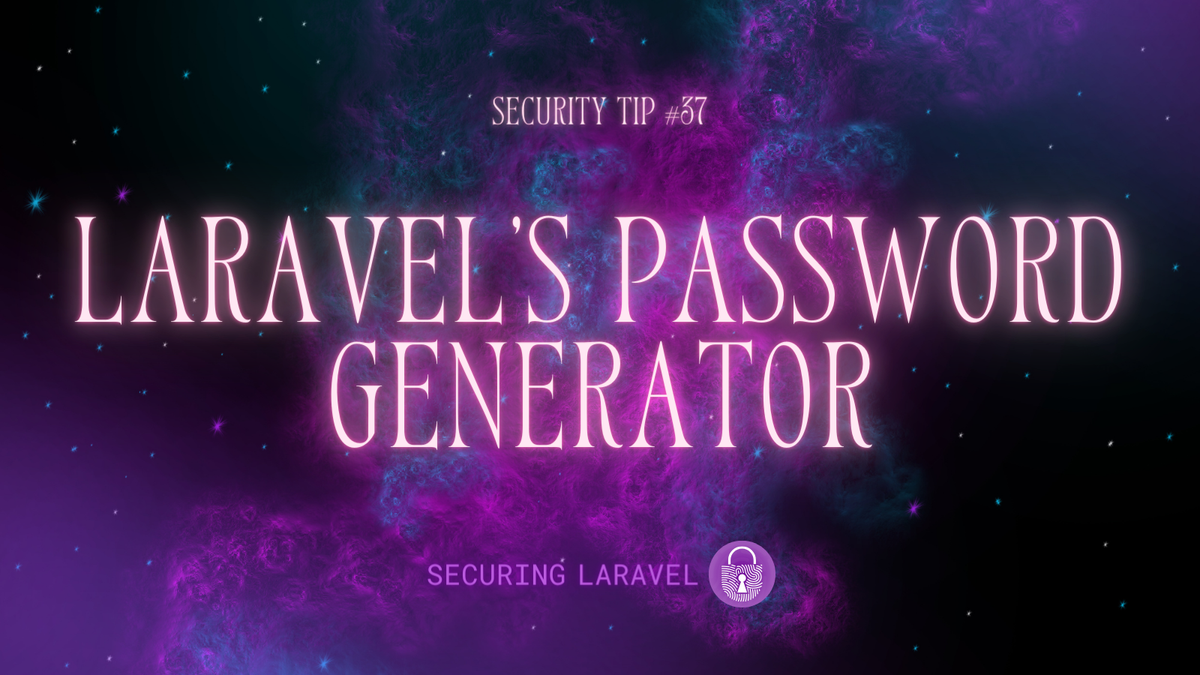
When generating new passwords, you need an algorithm that uses a cryptographically secure random generator (as per Security Tip: Cryptographically Secure Randomness) to ensure there is enough entropy to keep your passwords unguessable. A good way to do this is to generate lengthy passwords with a significant character set that includes lower case and uppercase letters, numbers, and extra symbols.
Laravel’s Password helper lets you do exactly that:
Str::password($length = 32, $letters = true, $numbers = true, $symbols = true, $spaces = false): string;
By default, the helper will return a 32 character incredibly secure password:
> $password = Str::password();
= "eY-j4B<kLf%o/k~x*#&9KUHPU8~!;I?8"
You can change the length, toggle on/off letters, numbers, symbols, and spaces:
> $password = Str::password(
length: 16,
letters: true,
numbers: false,
symbols: true,
spaces: true
);
= "$*|[# S*?/Qxj~W,"
Internally it uses random_int()
to securely build the password from it’s extensive character list, so it can be considered cryptographically secure.
So the next time you need to generate a password in your app, you can reach straight for Str::password()
.
Update (2024-05-09)
Since writing this article, I have built my own Random package, which offers an alternative to generating cryptographically secure passwords, but with more flexibility around character sets, etc. Check it out if Str::random()
doesn't meet your needs: https://github.com/valorin/random.
If you found this security tip useful, subscribe to get weekly Security Tips straight to your inbox. Upgrade to a premium subscription for exclusive monthly In Depth articles, or drop a coin in the tip jar to show your support.
When was the last time you had a penetration test? Book a Laravel Security Audit and Penetration Test, or a budget-friendly Security Review!
You can also connect with me on Bluesky, or other socials, and check out Practical Laravel Security, my interactive course designed to boost your Laravel security skills.