Security Tip: Laravel 11's Controller Authorisation & Validation Methods
[Tip#75] As part of the simplification of the app structure in Laravel 11, the Request Authorisation and Validation methods are no longer available on the controller - here's how you get it back.
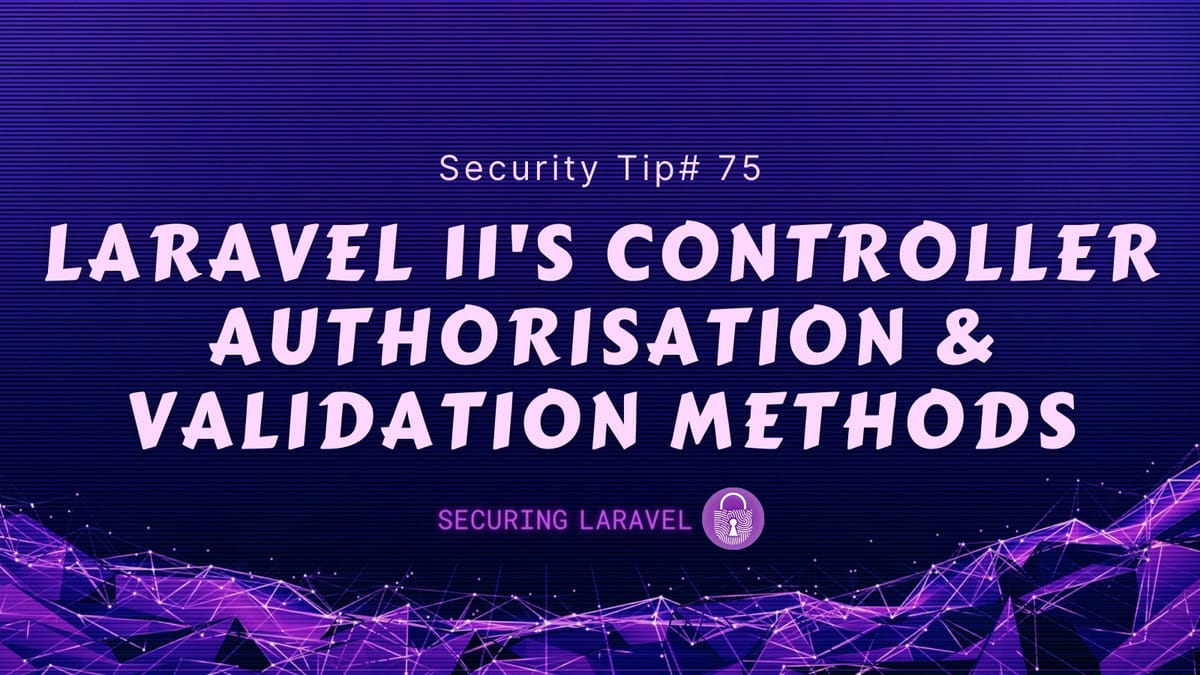
Next up in our series on Laravel 11’s security features, we’ve got the simplification of the base Controller
class, which no longer includes the AuthorizesRequests
and ValidatesRequests
traits by default.
The base controller included in new Laravel applications has been simplified. It no longer extends Laravel's internal Controller class, and theAuthorizesRequests
andValidatesRequests
traits have been removed, as they may be included in your application's individual controllers if desired:
<?php
namespace App\Http\Controllers;
abstract class Controller
{
//
}
This means that you can no longer use the following helpers in your controller actions, without first including the associated traits:
// ValidatesRequests
$this->validate();
// AuthorizesRequests
$this->authorize();
$this->authorizeResource();
Note, there are more helpers in the trait. I've just included the main ones.
If you’re like me and always pass a Illuminate\Http\Request
into your controller actions, and run $request->validate()
on that, and put all of your authorisation in your routes, then you might not notice this change.
However, if you use either of these methods, you’ll probably want to get them back when starting a new project. Especially if you put your authorisation inside your controllers - which a lot of folks do!
Anything that makes authorisation harder to access is going to increase missing authorisation vulnerabilities, although I do note the documentation has switched to recommending the use of the Gate
facade over this helper, so that may be a good direction to head instead.
But if you do need them, how do you get these back?
Simple, open up your app/Http/Controllers/Controller.php
file, and add in the traits:
<?php
namespace App\Http\Controllers;
use Illuminate\Foundation\Auth\Access\AuthorizesRequests;
use Illuminate\Foundation\Validation\ValidatesRequests;
abstract class Controller
{
use AuthorizesRequests;
use ValidatesRequests;
}
That’s basically it. 🙂
Ultimately it’s a pretty simple change with a trivial fix, but I felt it was worth noting as part of our series on security updates in Laravel 11, in case you rely on these methods and find them missing when building new projects.