Security Tip #76: Laravel 11's Automatic Password Rehashing
Let's check out three of the configuration options available as part of Automatic Password Rehashing: custom fields, disabling rehashing, and changing bcrypt rounds.
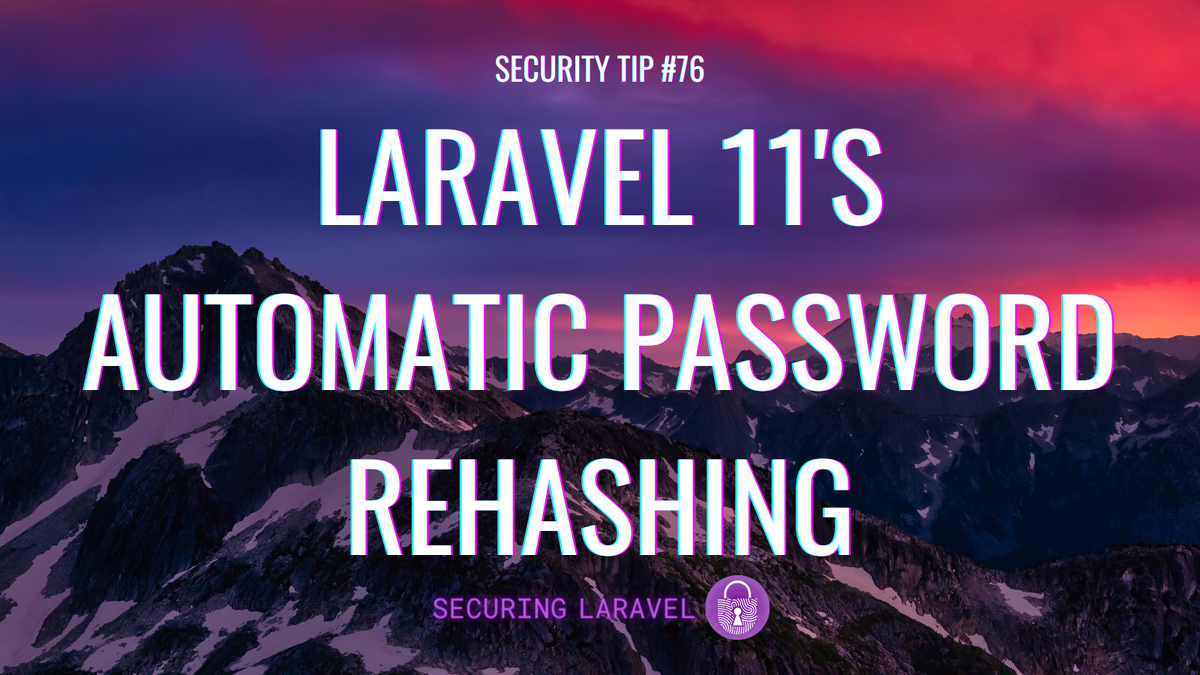
Next up in our series on Laravel 11’s security features, we have a rather familiar one: “Automatic Password Rehashing”.
Laravel's default password hashing algorithm is bcrypt. The "work factor" for bcrypt hashes can be adjusted via theconfig/hashing.php
configuration file or theBCRYPT_ROUNDS
environment variable.
Typically, the bcrypt work factor should be increased over time as CPU / GPU processing power increases. If you increase the bcrypt work factor for your application, Laravel will now gracefully and automatically rehash user passwords as users authenticate with your application.
We’ve covered this topic a few times before, so rather than cover the same ground, we’re going to look at the configuration options associated with it. I’ll include links to more information at the bottom if you’d like to dive deeper.
Disabling Automatic Rehashing
If you need to disable the automatic password rehashing functionality, you can toggle the rehash_on_login
flag in the config/hashing.php
file.
// config/hashing.php
'rehash_on_login' => false,
This may be useful if you’ve implemented your own authentication system or custom password handling, where there may not be passwords to rehash or fields may not be easy for Laravel to automatically identify.
Custom Password Fields
If your app stores passwords in a database field other than password
, you can tell Laravel name of your password field through the getAuthPasswordName()
method on your User model (which extends the Authenticatable
trait).
class User extends Authenticatable
{
// ...
public function getAuthPasswordName()
{
return 'user_password';
}
}
Laravel uses this method to identify the existing password hash, check if it needs to be rehashed, and if so, save the rehashed password.
Increase bcrypt Rounds
You can override the bcrypt rounds/work factor used when hashing passwords by setting BCRYPT_ROUNDS
or publishing and editing config/hashing.php
. Laravel 11 defaults to 12, which is a good default for most apps, but you can increase it if password security is a major concern.
// .env
BCRYPT_ROUNDS=12
// config/hashing.php
'bcrypt' => [
'rounds' => env('BCRYPT_ROUNDS', 12),
'verify' => env('HASH_VERIFY', true),
],
More Information: