Security Tip: Casting Request Values
[Tip #39] Why treat all user input as strings when you can pull out specific values and automatically cast them as the types you're expecting?
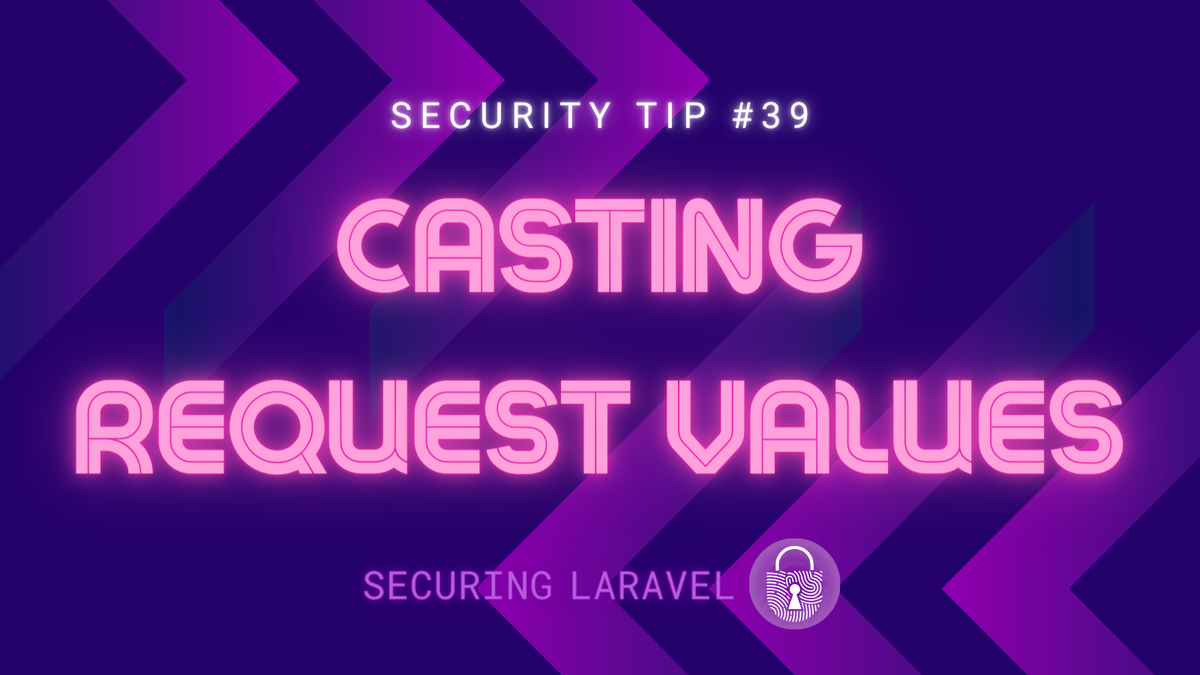
Laravel’s Request object (Illuminate\Http\Request
) includes a number of methods for extracting user input. My personal favourite is the validate()
method (see Security Tip: Don’t Trust User Input), however there are a number of others you can reach for instead, depending on your use case.
Sometimes you’ll need to pull out specific request values and transform them into specific types, such as integers or Booleans. Although you can do this manually, there is always the potential to forget or rely on type juggling and for subtle vulnerabilities to be introduced.
So instead, a safer way to do it is to ask the Request object to give you the input value in the type you need it in. It’ll return a properly typed value that you can use safely throughout your app.
The available methods are:
public function string($key, $default = null): \Illuminate\Support\Stringable;
public function boolean($key = null, $default = false): bool;
public function integer($key, $default = 0): int;
public function float($key, $default = 0.0): float;
public function date($key, $format = null, $tz = null): \Illuminate\Support\Carbon;
public function enum($key, $enumClass): <Enum>;
With the exception of string()
, they are all pretty self-explanatory.
The string()
method actually returns an instance of Illuminate\Support\Stringable
, which you can easily manipulate via a fluent interface.
You won’t need this all the time, but it’ll save you some effort and reduce potential bugs when you do. 🙂