Security Tip: Don’t Trust User Input!
[Tip #7] Always pass user input through a validator to ensure you only get the data you're expecting.
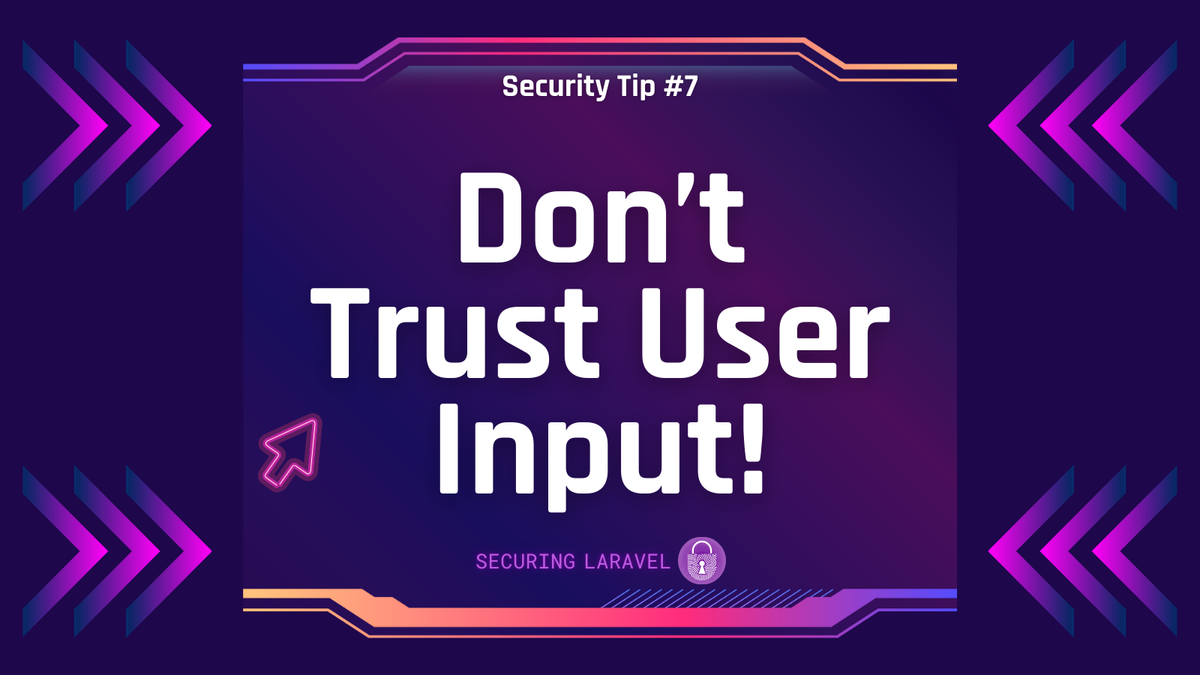
Don’t trust user input.
Don’t trust user input.
And one more for good measure…
Don’t trust user input.
You should always pass user input through a validator before you use it, and here are a few reasons why:
- It forces you to define explicit rules which state exactly what sort of input is allowed in each field.
- You’re far less likely to have unexpected data that causes your application to do unexpected things.
- You have control over which fields are passed into a model for mass-assignment.
- Your user interface can understand and display friendly errors to your users with minimal effort on your part.
- Cross-Site Scripting (XSS)
- SQL Injection (SQLi)
- And more...
Check out the docs for the many ways to use a validator in Laravel.
My preferred method is within controller actions on the Request
object, or using a FormRequest object for more complicated forms.
/**
* Store a new blog post.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$validated = $request->validate([
'title' => ['required', 'unique:posts', 'max:255'],
'body' => ['required', 'string'],
'publish_at' => ['nullable', 'date'],
]);
// $validated contains only valid user input
$post = Post::create($validated);
// ...
}
Go forth and validate all the things! 😎
Found this security tip helpful? Don't forget to subscribe to receive new Security Tips each week, and upgrade to a premium subscription to receive monthly In Depth articles, or toss a coin in the tip jar.
Reach out if you're looking for a Laravel Security Audit and Penetration Test or a budget-friendly Security Review, and find me on the various socials through Pinkary. Finally, don't forget to check out Practical Laravel Security, my interactive security course.