Security Tip: Use Route Groups!
[Tip#24] It may sound trivial, but it's easy to overlook.
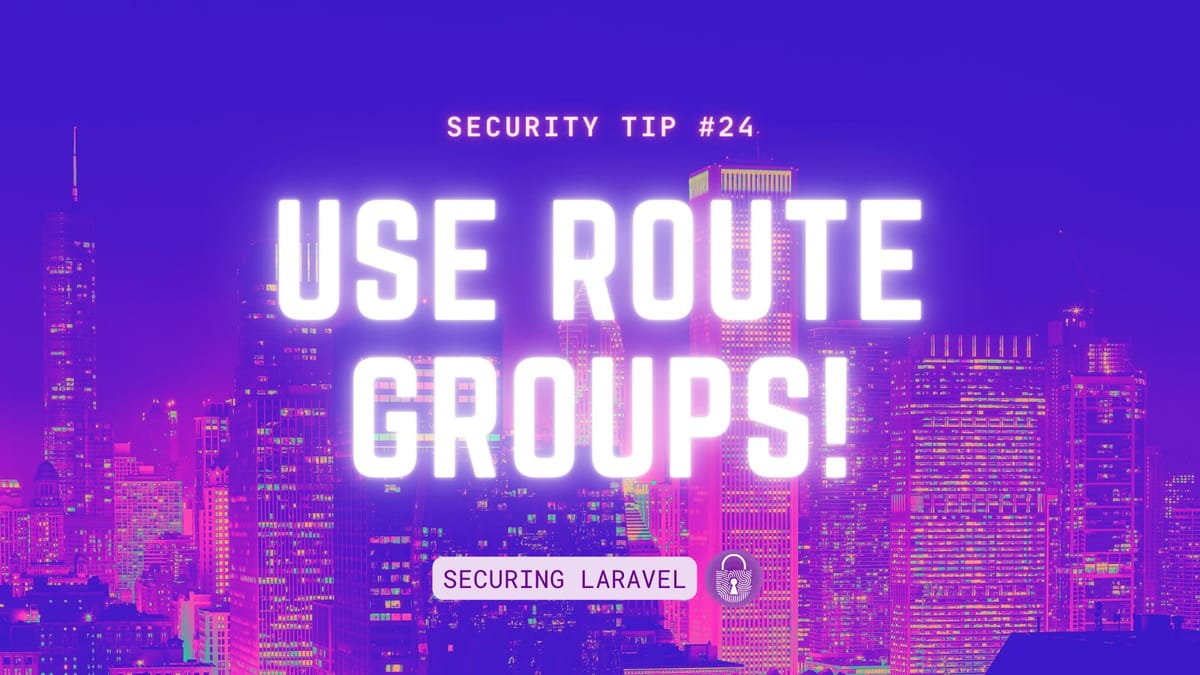
🤓 Learn to Think Like a Hacker with my hands-on practical security course: Practical Laravel Security! 🕵️
⚠️ Need a security audit but don't have the budget for a full penetration test? Book in a Laravel Security Review! 🕵️
Route Groups are awesome for so many reasons, and as you’d expected, one of those reasons is security. Not only do they make it easy to review the access requirements for each of your routes in the one place but they also serve as a fantastic reminder that you need to be aware of the permissions for each of your routes.
Consider this: when adding a new route, you’ll open up the routes file, and look for a suitable place to put it. If everything is grouped, you need to decide what access level that route needs when you add it. You’re unlikely to forget and your app stays secure.
Alternatively, if you leave your access control in your controllers, you’ll add your route somewhere, open up a blank controller and start coding… and sometimes forget about access control, leaving an endpoint wide open.
I recommend implementing as much of your access control as possible through middleware.
You’ve got the default auth
and guest
helpers for basic authentication checks, with Policy Objects to handle more complex rules, but if the app I’m working on requires something more complicated, I’ll implement some custom middleware to handle that logic too. That way it’s all encompassed within middleware and the route layer, making it harder for me to overlook and forget.
As a quick guide:
Authenticated Users Only
Route::middleware(['auth'])->group(function () {
// ...
});
Unauthenticated Guests Only
// Authenticated Users Only
Route::middleware(['guest'])->group(function () {
// ...
});
Authenticated With Specific Auth Guard Only
Route::middleware(['auth:admin'])->group(function () {
// ...
});
Authenticated & Product Policy Approved Only
Route::middleware(['auth'])->group(function () {
// ...
Route::middleware(['can:view,product'])->group(function () {
// ..
});
});
Authenticated & Custom Control
Route::middleware(['auth'])->group(function () {
// ...
Route::middleware(['editor'])->group(function () {
// ..
});
});
Useful Documentation: