Security Tip: Default Password Rules
[Tip #11] Why duplicate password validation rules across your app when you can define defaults once?
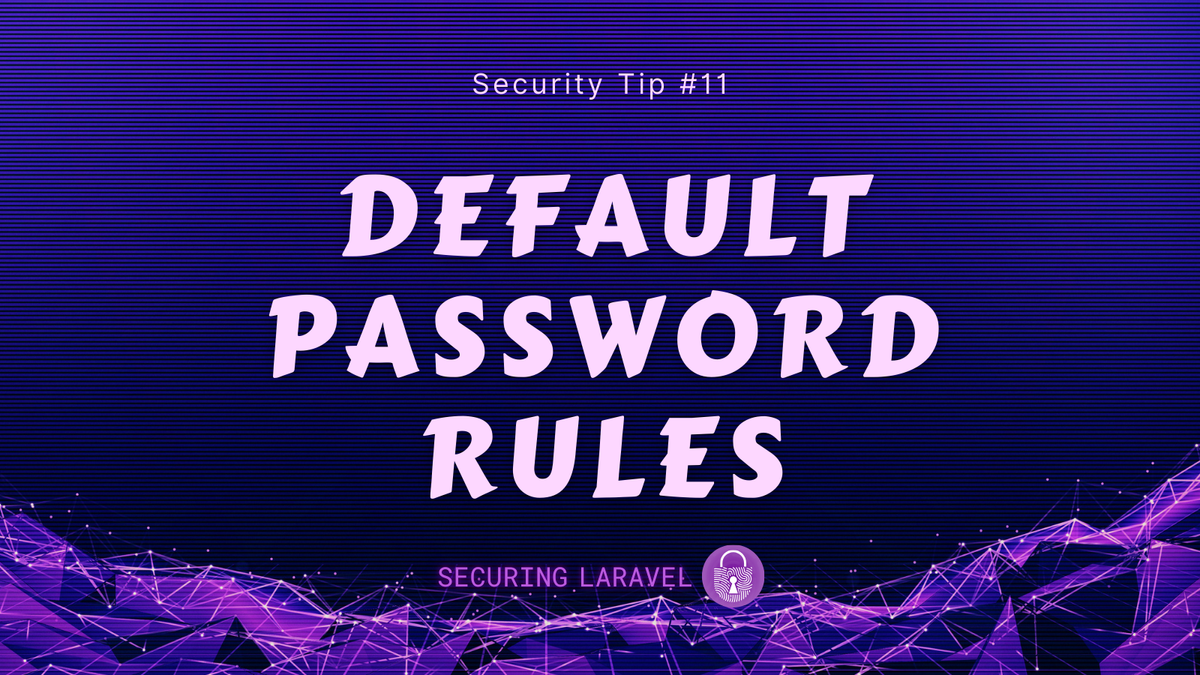
Password Rules are typically defined on a project or company level and then reused across an app, so you’ll probably find yourself copy-pasting the rules from one validator to another when building registration, password change, etc, and then if the rules ever change, you’ll have to bounce between all of the validators to update the rules. It doesn’t sound that hard, but it still takes effort, and if your app has multiple logins or registration forms, you’ll need to update and all of them…
Luckily for us, Laravel makes this easy!
Laravel’s Password Validator includes the concept of Default Password Rules. You use it by defining your password rules once, usually in a Service Provider, and then you can simply refer to these rules in each validator as Password::defaults()
.
Let’s define a basic minimum length:
use Illuminate\Validation\Rules\Password;
class AppServiceProvider extends ServiceProvider
{
// ...
public function boot()
{
Password::defaults(fn () => Password::min(8));
}
}
The validator can then look like this:
'password' => ['required', Password::defaults()],
Now we can change our password rules, and the validator won’t change at all.
Let's add in the uncompromised()
rule, which checks the submitted password against Have I Been Pwned's Pwned Passwords list.
Let's also only enable it on production:
use Illuminate\Validation\Rules\Password;
class AppServiceProvider extends ServiceProvider
{
public function boot()
{
Password::defaults(function () {
$rule = Password::min(8);
return $this->app->isProduction()
? $rule->uncompromised()
: $rule;
});
}
}
Done!
No changes needed for the validation rules in each controller/request. 😎
Found this security tip helpful? Don't forget to subscribe to receive new Security Tips each week, and upgrade to a premium subscription to receive monthly In Depth articles, or toss a coin in the tip jar.
Reach out if you're looking for a Laravel Security Audit and Penetration Test or a budget-friendly Security Review, and find me on the various socials through Pinkary. Finally, don't forget to check out Practical Laravel Security, my interactive security course.